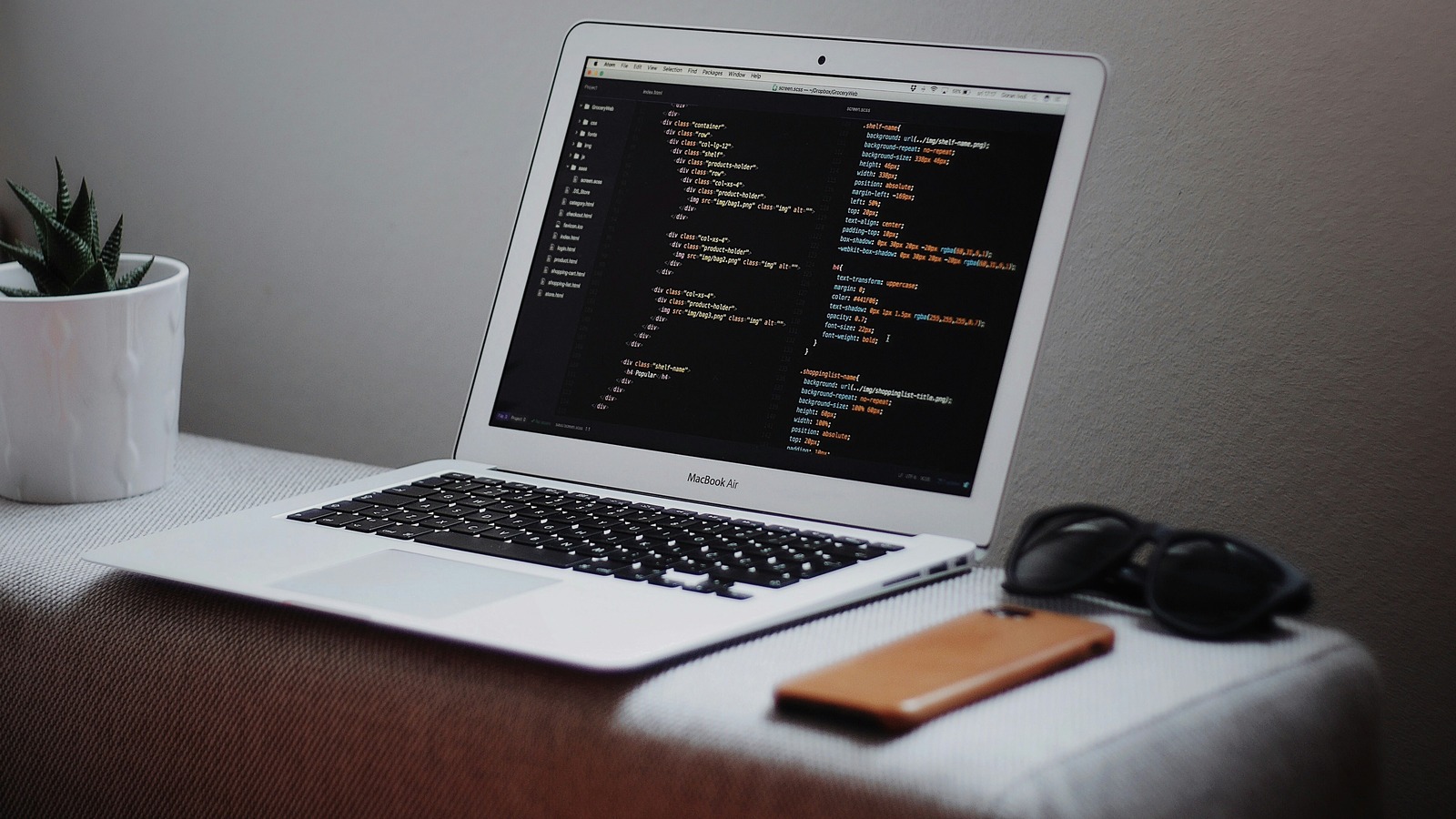
How to Subscribe to Webhooks with PHP using WaAPI
Webhooks are a powerful way to receive real-time updates from external services. In this guide, we'll walk you through the process of subscribing to webhooks using PHP and the WaAPI (WhatsApp API) service. Let's get started!
Prerequisites
- PHP 7.4 or higher installed on your server
- A WaAPI account (sign up at https://waapi.app)
- Your WaAPI instance ID and API token
- A publicly accessible server to receive webhook events
Step 1: Set Up Your PHP Environment
First, make sure you have a PHP server set up to receive incoming webhook requests. You can use frameworks like Laravel or Symfony, or a simple PHP script for this purpose.
Step 2: Create a Webhook Endpoint
Create a new PHP file named webhook_handler.php
and add the following code:
<?php
// Retrieve the request body
$payload = file_get_contents('php://input');
// Parse the JSON payload
$data = json_decode($payload, true);
// Verify the webhook signature (implementation depends on your webhook provider)
// For WaAPI, you can use the 'x-waapi-hmac' header
$signature = $_SERVER['HTTP_X_WAAPI_HMAC'] ?? '';
$secret = 'your_webhook_secret'; // Replace with your actual secret
if (!verifySignature($payload, $signature, $secret)) {
http_response_code(401);
exit('Invalid signature');
}
// Process the webhook data
processWebhook($data);
// Respond to the webhook
http_response_code(200);
echo 'Webhook received successfully';
function verifySignature($payload, $signature, $secret)
{
$expectedSignature = hash_hmac('sha256', $payload, $secret);
return hash_equals($expectedSignature, $signature);
}
function processWebhook($data)
{
// Implement your webhook processing logic here
// For example, you can log the data or trigger specific actions based on the event type
error_log('Received webhook: ' . print_r($data, true));
}
This script does the following:
- Retrieves the raw payload from the request body
- Parses the JSON payload into a PHP array
- Verifies the webhook signature to ensure the request is legitimate
- Processes the webhook data (you'll implement your custom logic here)
- Responds to the webhook request with a 200 OK status
Step 3: Configure Your WaAPI Instance to Send Webhooks
Now that you have a webhook endpoint set up, you need to configure your WaAPI instance to send webhooks to this endpoint. You can do this using the WaAPI dashboard or via the API.
Here's an example of how to set up the webhook URL using PHP and the WaAPI API:
<?php
$instanceId = 123; // Replace with your instance ID
$apiToken = 'your-api-token'; // Replace with your API token
$webhookUrl = 'https://your-server.com/webhook_handler.php'; // Replace with your webhook URL
$curl = curl_init();
curl_setopt_array($curl, [
CURLOPT_URL => "https://waapi.app/api/v1/instances/{$instanceId}/webhook",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => json_encode([
'url' => $webhookUrl
]),
CURLOPT_HTTPHEADER => [
"accept: application/json",
"authorization: Bearer {$apiToken}",
"content-type: application/json"
],
]);
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
This script sets up the webhook URL for your WaAPI instance. Make sure to replace the placeholders with your actual instance ID, API token, and webhook URL.
Step 4: Handle Webhook Events
Now that you've set up your webhook endpoint and configured WaAPI to send events to it, you need to implement the logic to handle these events. In the processWebhook
function of your webhook_handler.php
file, add code to handle different types of events:
function processWebhook($data)
{
$eventType = $data['event'] ?? '';
switch ($eventType) {
case 'message':
handleNewMessage($data);
break;
case 'status_change':
handleStatusChange($data);
break;
// Add more cases for other event types
default:
error_log('Unknown event type: ' . $eventType);
}
}
function handleNewMessage($data)
{
$message = $data['message'] ?? [];
$sender = $message['from'] ?? '';
$content = $message['body'] ?? '';
// Process the new message
error_log("New message from {$sender}: {$content}");
// Add your custom logic here (e.g., store in database, send a reply, etc.)
}
function handleStatusChange($data)
{
$newStatus = $data['status'] ?? '';
$instanceId = $data['instanceId'] ?? '';
// Process the status change
error_log("Instance {$instanceId} status changed to: {$newStatus}");
// Add your custom logic here (e.g., notify admins, update database, etc.)
}
Best Practices and Considerations
- Security: Always verify the webhook signature to ensure the requests are coming from a legitimate source.
- Idempotency: Design your webhook handler to be idempotent, as some events may be sent multiple times due to network issues.
- Async Processing: For time-consuming tasks, consider using a job queue to process webhook events asynchronously.
- Error Handling: Implement robust error handling and logging to troubleshoot issues with webhook processing.
- Rate Limiting: Be prepared to handle a high volume of webhook events and implement rate limiting if necessary.
Conclusion
You've now learned how to subscribe to webhooks using PHP and the WaAPI service. This powerful combination allows you to receive real-time updates from WhatsApp and integrate them into your application. Remember to handle webhook events securely and efficiently to make the most of this real-time data.
For more advanced features and detailed API documentation, refer to the official WaAPI documentation.