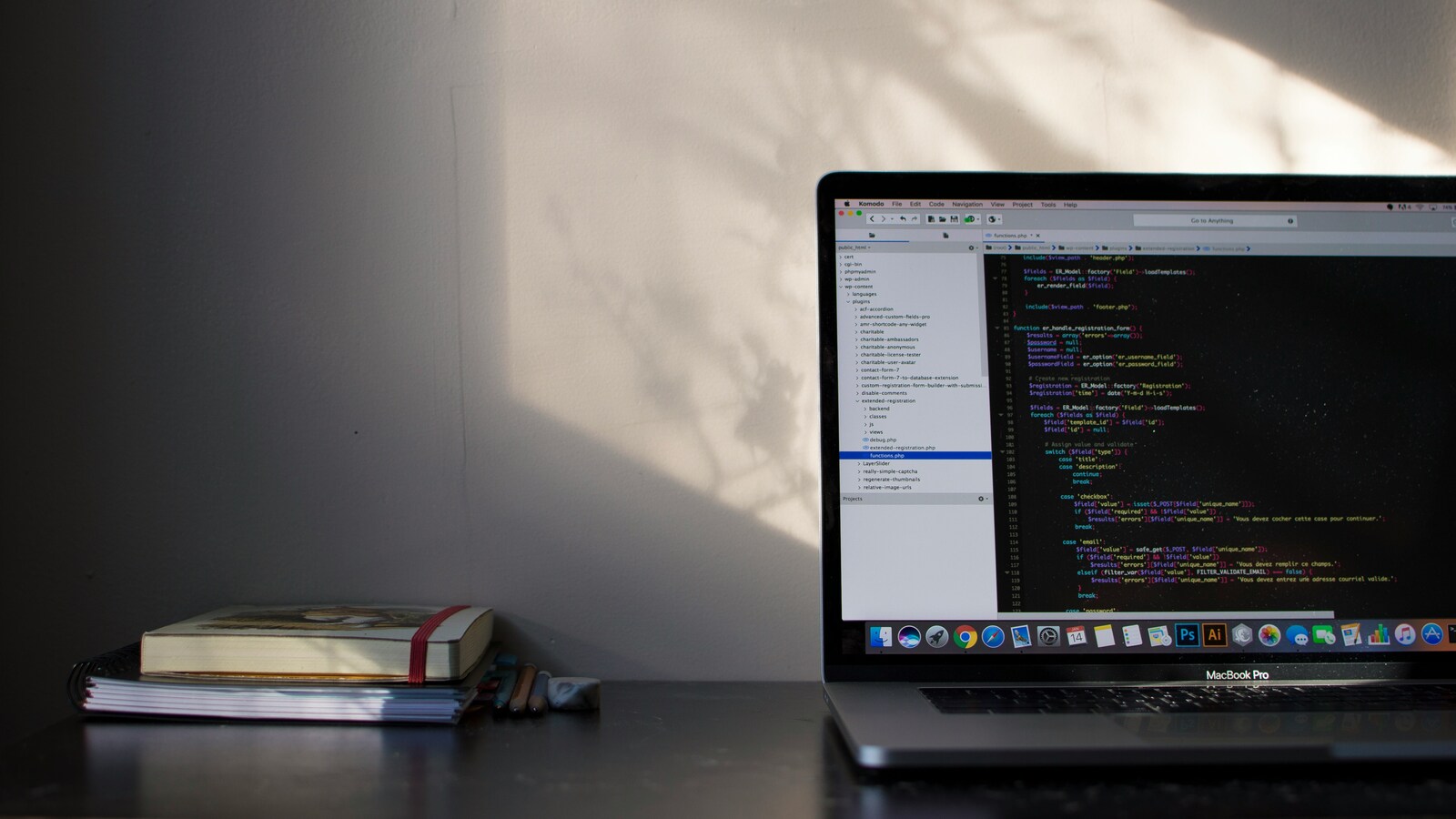
How to Send Bulk WhatsApp Messages with Python and WaAPI
Sending bulk messages on WhatsApp can be a powerful tool for businesses and developers. In this guide, we'll walk you through the process of sending bulk WhatsApp messages using Python and the WaAPI (WhatsApp API) service. Let's get started!
Prerequisites
- Python 3.x installed on your system
- A WaAPI account (sign up at https://waapi.app)
- Your WaAPI instance ID and API token
Step 1: Set Up Your Python Environment
First, let's set up our Python environment and install the required library. We'll be using the requests
library to make HTTP requests to the WaAPI.
Open your terminal and run the following command to install the requests
library:
pip install requests
Step 2: Import Required Libraries and Set Up API Credentials
Create a new Python file named bulk_whatsapp_sender.py
and add the following code:
import requests
import time
# Your WaAPI credentials
instance_id = 123 # Replace with your instance ID
api_token = "your-api-token" # Replace with your API token
# API endpoint
url = "https://waapi.app/api/v1/instances/{instance_id}/client/action/send-message"
# Headers for the API request
headers = {
"accept": "application/json",
"content-type": "application/json",
"authorization": "Bearer {api_token}"
}
Make sure to replace instance_id
and api_token
with your actual WaAPI credentials.
Step 3: Create a Function to Send a Single Message
Let's create a function that sends a single message to a given phone number:
def send_message(phone_number, message):
payload = {
"chatId": "{phone_number}@c.us",
"message": message
}
response = requests.post(url, json=payload, headers=headers)
if response.status_code == 200:
print("Message sent successfully to {phone_number}")
else:
print("Failed to send message to {phone_number}. Error: {response.text}")
Step 4: Implement Bulk Message Sending
Now, let's create a function to send bulk messages to multiple recipients:
def send_bulk_messages(recipients, message):
for phone_number in recipients:
send_message(phone_number, message)
time.sleep(15) # Wait for 15 seconds between messages to avoid rate limiting
# Example usage
recipients = ["1234567890", "9876543210", "5555555555"]
message = "Hello! This is a bulk message sent using Python and WaAPI."
send_bulk_messages(recipients, message)
In this function, we iterate through the list of recipients and send the same message to each one. We've added a 15-second delay between messages to avoid potential rate limiting issues.
Step 5: Run the Script
Save your bulk_whatsapp_sender.py
file and run it using Python:
python bulk_whatsapp_sender.py
This will send the specified message to all the phone numbers in the recipients
list.
Best Practices and Considerations
- Rate Limiting: Be mindful of WaAPI's rate limits. The 15-second delay between messages is a conservative approach. You may need to adjust this based on your specific plan and requirements.
- Error Handling: Implement more robust error handling in production code to manage potential API issues or network errors.
- Personalization: Consider personalizing messages for each recipient by using a template and filling in specific details for each user.
- Compliance: Ensure you have the necessary permissions to send bulk messages and comply with relevant regulations and WhatsApp's policies.
Conclusion
You've now learned how to send bulk WhatsApp messages using Python and the WaAPI service. This powerful combination allows you to automate your WhatsApp communication efficiently. Remember to use this capability responsibly and in compliance with all applicable rules and regulations.
For more advanced features and detailed API documentation, refer to the official WaAPI documentation.